Picture by Creator
Â
As a knowledge scientist, you want Python for detailed knowledge evaluation, knowledge visualization, and modeling. Nevertheless, when your knowledge is saved in a relational database, you want to use SQL (Structured Question Language) to extract and manipulate the information. However how do you combine SQL with Python to unlock the total potential of your knowledge?
On this tutorial, we are going to be taught to mix the facility of SQL with the flexibleness of Python utilizing SQLAlchemy and Pandas. We are going to learn to connect with databases, execute SQL queries utilizing SQLAlchemy, and analyze and visualize knowledge utilizing Pandas.
Set up Pandas and SQLAlchemy utilizing:
pip set up pandas sqlalchemy
Â
1. Saving the Pandas DataFrame as an SQL Desk
Â
To create the SQL desk utilizing the CSV dataset, we are going to:
- Create a SQLite database utilizing the SQLAlchemy.
- Load the CSV dataset utilizing the Pandas. The countries_poluation dataset consists of the Air High quality Index (AQI) for all nations on the earth from 2017 to 2023.Â
- Convert all of the AQI columns from object to numerical and drop row with lacking values.
# Import needed packages
import pandas as pd
import psycopg2
from sqlalchemy import create_engine
# creating the brand new db
engine = create_engine(
"sqlite:///kdnuggets.db")
# learn the CSV dataset
knowledge = pd.read_csv("/work/air_pollution new.csv")
col = ['2017', '2018', '2019', '2020', '2021', '2022', '2023']
for s in col:
knowledge[s] = pd.to_numeric(knowledge[s], errors="coerce")
knowledge = knowledge.dropna(subset=[s])
Â
- Save the Pandas dataframe as a SQL desk. The `to_sql` operate requires a desk identify and the engine object. Â
# save the dataframe as a SQLite desk
knowledge.to_sql('countries_poluation', engine, if_exists="replace")
Â
Because of this, your SQLite database is saved in your file listing.Â
Â
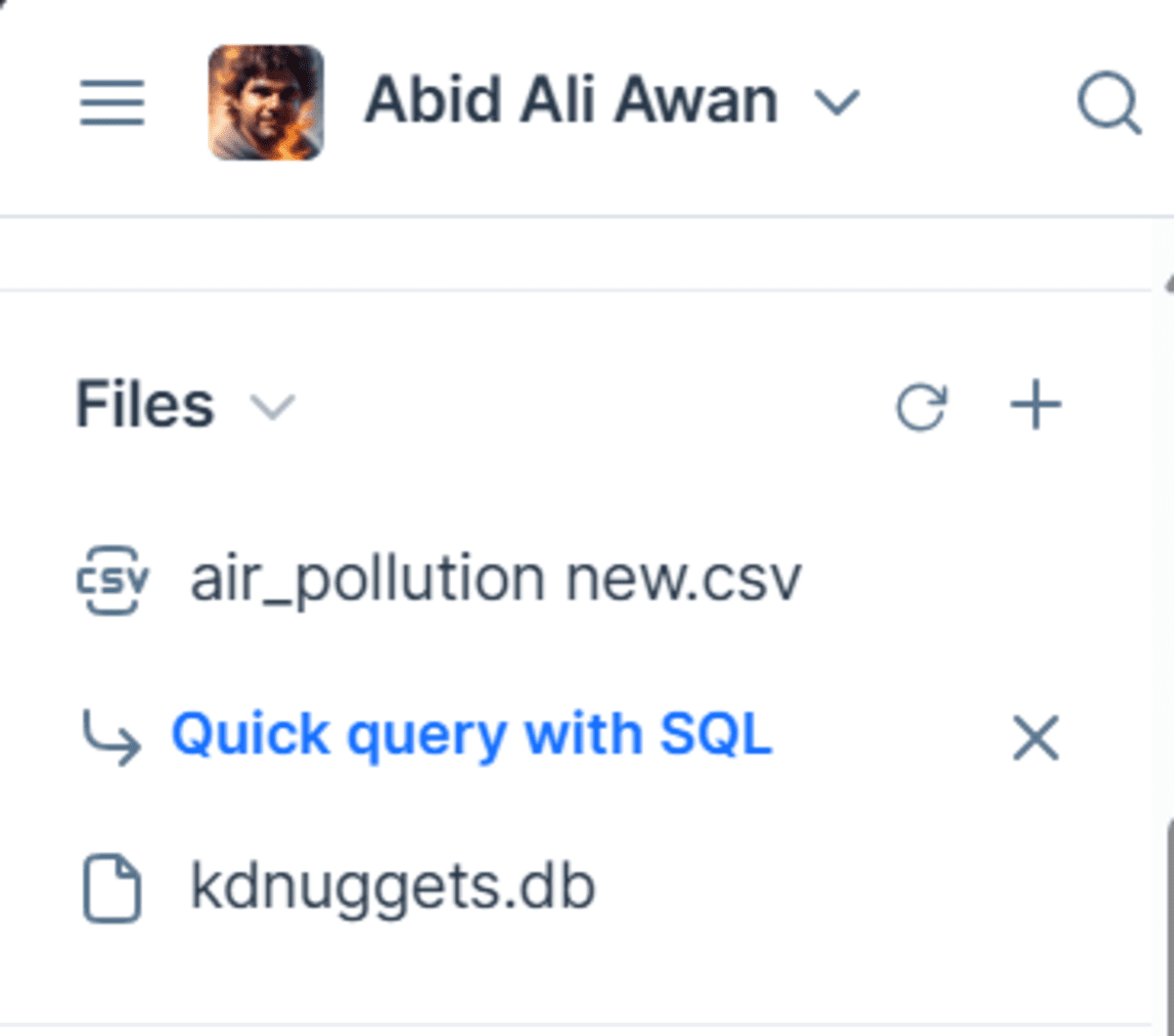
Â
Notice: I’m utilizing Deepnote for this tutorial to run the Python code seamlessly. Deepnote is a free AI Cloud Pocket book that may provide help to shortly run any knowledge science code.Â
Â
2. Loading the SQL Desk utilizing Pandas
Â
To load your entire desk from the SQL database as a Pandas dataframe, we are going to:
- Set up the reference to our database by offering the database URL.
- Use the `pd.read_sql_table` operate to load your entire desk and convert it right into a Pandas dataframe. The operate requires desk anime, engine objects, and column names.Â
- Show the highest 5 rows.Â
import pandas as pd
import psycopg2
from sqlalchemy import create_engine
# set up a reference to the database
engine = create_engine("sqlite:///kdnuggets.db")
# learn the sqlite desk
table_df = pd.read_sql_table(
"countries_poluation",
con=engine,
columns=['city', 'country', '2017', '2018', '2019', '2020', '2021', '2022',
'2023']
)
table_df.head()
Â
The SQL desk has been efficiently loaded as a dataframe. This implies that you may now use it to carry out knowledge evaluation and visualization utilizing in style Python packages comparable to Seaborn, Matplotlib, Scipy, Numpy, and extra.
Â

Â
3. Working the SQL Question utilizing Pandas
Â
As an alternative of proscribing ourselves to 1 desk, we will entry your entire database by utilizing the `pd.read_sql` operate. Simply write a easy SQL question and supply it with the engine object.
The SQL question will show two columns from the “countries_population” desk, kind it by the “2023” column, and show the highest 5 outcomes.
# learn desk knowledge utilizing sql question
sql_df = pd.read_sql(
"SELECT city,[2023] FROM countries_poluation ORDER BY [2023] DESC LIMIT 5",
con=engine
)
print(sql_df)
Â
We bought to the highest 5 cities on the earth with the worst air high quality.Â
metropolis 2023
0 Lahore 97.4
1 Hotan 95.0
2 Bhiwadi 93.3
3 Delhi (NCT) 92.7
4 Peshawar 91.9
Â
4. Utilizing the SQL Question Outcome with Pandas
Â
We are able to additionally use the outcomes from SQL question and carry out additional evaluation. For instance, calculate the common of the highest 5 cities utilizing Pandas.Â
average_air = sql_df['2023'].imply()
print(f"The average of top 5 cities: {average_air:.2f}")
Â
Output:
The common of prime 5 cities: 94.06
Â
Or, create a bar plot by specifying the x and y arguments and the kind of plot.Â
sql_df.plot(x="city",y="2023",variety = "barh");
Â

Â
Conclusion
Â
The probabilities of utilizing SQLAlchemy with Pandas are infinite. You’ll be able to carry out easy knowledge evaluation utilizing the SQL question, however to visualise the outcomes and even prepare the machine studying mannequin, you must convert it right into a Pandas dataframe.Â
On this tutorial, we now have realized methods to load a SQL database into Python, carry out knowledge evaluation, and create visualizations. If you happen to loved this information, additionally, you will recognize ‘A Information to Working with SQLite Databases in Python‘, which gives an in-depth exploration of utilizing Python’s built-in sqlite3 module.
Â
Â
Abid Ali Awan (@1abidaliawan) is an authorized knowledge scientist skilled who loves constructing machine studying fashions. At present, he’s specializing in content material creation and writing technical blogs on machine studying and knowledge science applied sciences. Abid holds a Grasp’s diploma in know-how administration and a bachelor’s diploma in telecommunication engineering. His imaginative and prescient is to construct an AI product utilizing a graph neural community for college kids fighting psychological sickness.